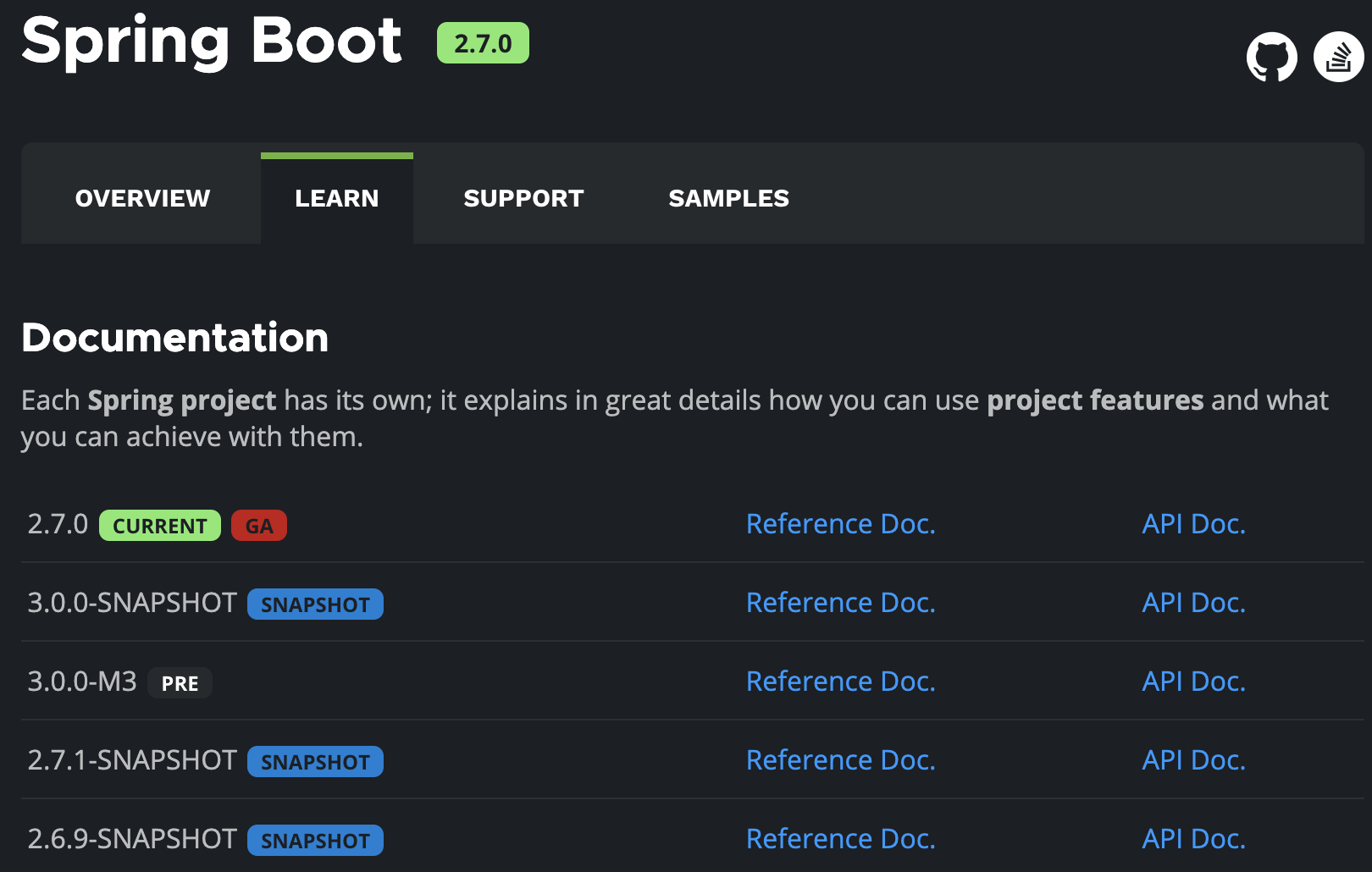
출처
[무료] 스프링 입문 - 코드로 배우는 스프링 부트, 웹 MVC, DB 접근 기술 - 인프런 | 강의
스프링 입문자가 예제를 만들어가면서 스프링 웹 애플리케이션 개발 전반을 빠르게 학습할 수 있습니다., - 강의 소개 | 인프런...
www.inflearn.com
스프링을 배우는 이유는?
스프링을 배우는 이유는 여러 가지가 있겠지만 실무에서 제대로 동작하는 애플리케이션을 만들기 위해서다.
1. 강좌의 순서
- 프로젝트 생성
- 웹 서버 실행
- 회원 도메인 개발
- 웹 MVC 개발
- DB 연동 JDBC, JPA, 스프링 데이터 JPA
- 테스트 케이스 작성
2. 사용 기술
-SpringBoot
- JPA
- Gradle
- HIBERNATE
JPA의 구현체.
ORM 프레임워크.
SQL을 직접 사용하지 않고, 매서드 호출만으로 쿼리 수행. (다만 SQL 작성보다 성능이 좋지는 않음)
- Thymeleaf
Thymeleaf (타임리프)
컨트롤러가 전달하는 데이터를 이용하여 동적으로 화면을 구성할 수 있게 해 준다. html 태그를 기반으로 th:속성을 이용하여 동적인 View를 제공한다. 웹에서 가장 기본이 되는 HTML로 진입장벽이 낮고 쉽게 배울 수 있다는 장점이 있다.
Thymeleaf 디펜던시 추가
1. Maven(pom.xml)
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
2. Gradle(build.gradle)
dependencies {
...
implementation'org.springframework.boot:spring-boot-starter-thymeleaf'
}
3. 프로젝트 생성
https://start.spring.io/
Gradle Project, Java, 2.7.0, Java version은 11, Dependencies는 Spring Web과 Thymeleaf를 선택한다.
예전에는 Maven Project를 주로 선택했지만 요즘에는 Gradle Project를 더 선호한다.
- 빌드 시에 오류가 뜨면 한번 확인해볼 만한 사항
intelliJ 환경설정에서 빌드, 실행, 배포 -> 빌드 도구 -> Gradle -> 다음을 사용하여 빌드 및 실행 : IntelliJ IDEA로 변경 (테스트도 동일)
4.1 주요 라이브러리
spring-boot-starter-web
spring-boot-starter-tomcat : 톰캣(웹 서버)
spring-webmvc : 스프링 웹 MVC
spring-boot-starter-thymeleaf : 타임리프 템플릿 엔진(View)
spring-boot-starter (공통) : 스프링 부트 + 스프링 코어 + 로깅
spring-boot
spring-core
spring-boot-starter-logging
logback, slf4j -> 이 2개의 조합을 많이 사용한다. 로그로 남겨야 지속적인 관리가 가능하다.
4.2 테스트 라이브러리
spring-boot-starter-test
Junit : 테스트 프레임 워크
mockito : 목 라이브러리
assertj : 테스트 코드를 좀 더 편하게 작성할 수 있도록 도와주는 라이브러리
spring-test : 스프링 통합 테스트 지원
5. main -> resorces -> static 폴더에 index.html 파일을 생성하면 첫 화면(웰컴 페이지)이 된다.
6. 참조 문서
6.1 SpringBoot Reference
Spring makes Java simple.
Level up your Java code and explore what Spring can do for you.
spring.io
projects -> springboot -> learn
해당 버전의 Reference Doc을 클릭하면 스프링 부트 참조 문서를 확인할 수 있다.
6.2 thymeleaf Reference
Thymeleaf
Integrations galore Eclipse, IntelliJ IDEA, Spring, Play, even the up-and-coming Model-View-Controller API for Java EE 8. Write Thymeleaf in your favourite tools, using your favourite web-development framework. Check out our Ecosystem to see more integrati
www.thymeleaf.org
Docs 참조
7. HelloController (<- Web Application의 첫 번째 진입점은 Controller)
// Hello Controller
//...
@GetMapping("hello") // http://localhost:8080/hello
public String hello(Model model) {
model.addAttribute("data", "hello!! 되나요?");
return "Hello"; // hello.html 로딩
}
<!-- hello.html -->
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>Title</title>
<meta http-equiv="Content-Type" content="text/html" charset="UTF-8" />
</head>
<body>
<p th:text="'안녕하세요! ' + ${data}">안녕하세요 손님</p>
</body>
</html>
<프로젝트 결과 값>
<동작 환경>
- Controller에서 return 값으로 문자를 반환하면 viewResolver(뷰 리졸버)가 화면을 찾아서 처리한다.
- 스프링 부트 템플릿 엔진 기본 viewName 매핑
- 'resources:templates/' + {viewName} + '.html'
참고 : 'spring-boot-devtools' 라이브러리를 추가하면, 'html'파일을 컴파일만 해주면 서버 재시작 없이 view 파일 변경이 가능하다.
인텔리 J 컴파일 방법 : 메뉴 -> build -> Recompile
'programming > SpringBoot' 카테고리의 다른 글
[Spring] 스프링 입문강의 3 (회원 관리 예제) (0) | 2022.06.25 |
---|---|
[Spring] 스프링 입문 강의 2 (콘텐츠의 종류 - 정적 콘텐츠, MVC, API) (0) | 2022.06.24 |
새로운 프로젝트 시작! (0) | 2022.04.28 |
[SpringBoot] EC2에 프로젝트 clone 받기 (0) | 2022.03.01 |
[SpringBoot] 롬복 (0) | 2021.11.24 |